STEVE DEWHURST | C++
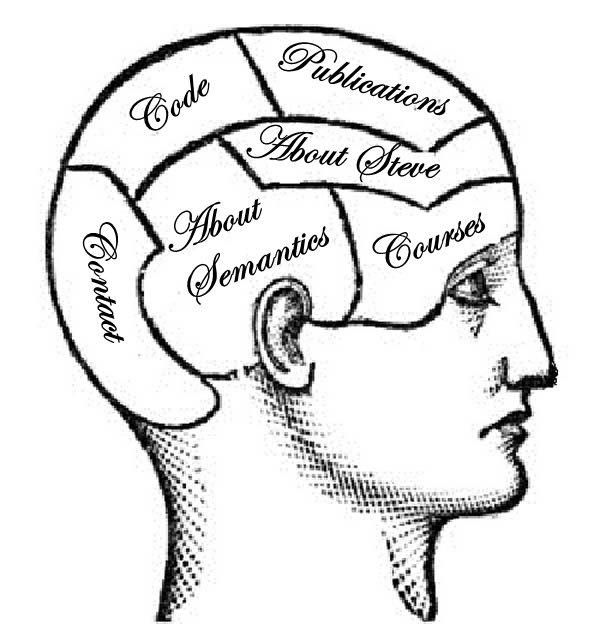
Once, Weakly
28 March 2017: Variadic CRTP
The Curiously-Recurring Template Pattern (CRTP) is a pleasant and common C++ coding idiom. This installment of Once, Weakly combines CRTP with variadic template template parameter packs to implement restricted numeric types suitable for implementing memory-mapped devices.Variadic CRTP [pdf]
crtp_components.h
number.h
writeonly.h
main.cpp
15 February 2017: Template Trees for Modern C++ Interfaces
Modern C++ increasingly uses “Do What I Mean” (DWIM) interfaces that depend on interface selection based on secondary properties of types. Often, these interfaces make use of SFINAE techniques and/or static assertions that require the ability to compose complex compile time queries about types.This installment of Once, Weakly describes the implementation and use of a simple facility for compile time composition and evaluation of abstract syntax trees (ASTs) of template predicates.
Template Trees for Modern C++ Interfaces [pdf]
pred_expr.h
pred_expr_traits.h
stl_iterator_traits.h
shape.h
main.cpp
29 February 2016: Talking to Typelists
Type sequences implemented with variadic templates have largely supplanted the traditional use of typelists in new C++ code. However, we have nearly two decade's worth of complex, debugged, and useful legacy typelist code that we’d like to leverage for use with type sequences without having to rewrite them.Additionally, modern C++ metaprogramming makes extensive use of index sequences as well as type sequences. Many index sequence meta‐algorithms are logically similar to corresponding meta‐algorithms on typelists (and type sequences). Wouldn't it be convenient to simply and automatically convert a typelist meta‐algorithm into a functionally‐similar index sequence meta‐algorithm?
That's what we’re going to do in this installment of Once, Weakly.
Talking to Typelists [pdf]
Talking to Typelists [html]
typelist03.h
typelist1114.h
index_sequence.h
sequence_conversions.h
Note: the items on "C++ommon Knowledge" that appeared in this space between April and August of 2004 are now available as C++ Common Knowledge: Essential Intermediate Programming.
21 February 2004: Typelist Meta-Algorithm Implementation Tricks
We examine a few more typelist meta-algorithms to motivate a few somewhat half-baked metaprogramming techniques used to implement them. (That’s why I’m calling them “tricks” instead of something more pretentious, like “strategies.”)23 December 2003: Checked Bridge Protopattern
A (proto)pattern that allows different versions of Bridge interfaces and implementations to work together. When the Bridge pattern is used with different versions of software that are distributed at different times, it is often the case that the interface part of the Bridge may be paired with an implementation part that was developed for an earlier or later version of the interface. The Checked Bridge pattern shows how to export the implementation requirements of an interface to potential implementations, and perform a fine-grain capability query to ensure that a particular aspect of an interface’s functionality is supported by its implementation.9
September 2003: Typelist Meta-Algorithms
How to perform compile time partition, sorting,
and transform of typelists, with accompanying meta-predicates,
meta-comparitors, and meta-function object adapters.
1 April 2003: A Matter of Judgement
I defend myself against the forces of zealotry. Along the way we discuss using non-standard switches to implement coroutines and playing with dolls.18 March 2003: Introduction to Traits
An introduction to a basic template programming technique.11 March 2003: What Are You, Anyway?
We look at one of the more obsure syntactic problems of template programming: how to guide the compiler's parse when there is not enough information available for the compiler to do the job on its own. Along the way we discuss and disambiguate the "rebind" mechanism employed by the standard containers.3 March 2003: Attack of the Clones
This item looks at the utility of implementing a "clone" operation on a hierarchy, and discusses its implications for designing frameworks and ordering a meal in a Swedish restaurant.1 March 2003: A Problem in Coordination
This item looks at a problem in class template instantiation interface design that plays off safety versus flexibility.23 February 2003: You Dis'n Me?
We discuss design of a framework for the dismantling of an arbitrary type into its component parts, and its later regeneration for the purpose of compile time type manipulation. We also show how to extend the framework to include arbitrarily fine detail on type-related information. We then show how some simple pattern matching and substitution and replacement can be performed on a dismantled type before a normal type is regenerated from it. In effect, we now have two equivalent, but structurally distinct, versions of the same type. The normal version is optimized for use in a traditional fashion: accessing its operations, causing code to be generated that will execute at runtime, etc. The dismantled version is optimized for compile time analysis and manipulation. Therefore, we now have the ability to move an arbitrary type between representations according to how we want to use it. The invertibility of the representations assures us that either representation will contain all the information present in the other.6 January 2003: Template Dismantling
It's not uncommon in template metaprogramming to dismantle a type into its component parts and put it back together differently. In this episode, we'll do the same thing with types that are generated from templates. We'll first show how to determine whether a given type was generated from a template. Then we'll see how to work back from the instantiated type to the individual components of its instantiation (including the instantiated template itself), mix them up, replace them, tweak them, and produce a new type.3 January 2003: Unrolling Expertise
We discuss the use of template metaprogramming to improve efficiency while keeping the users of our facility in happy ignorance of what's going on behind the scenes. Along the way we'll look at properly general generic algorithms, automated loop unrolling, algorithm selection based on whether arguments are integer constant-expressions, and the beneficial aspects of ignorance.6 December 2002: Type Structures
We examine the use of "type-structures" as a compile time analog of runtime data structures. We discuss how to construct and manipulate at compile time simple lists of integers, function pointers, types, and templates.24 November 2002: SFINAE Sono Buoni
We examine the concept of SFINAE (substitution failure is not an error). This fairly straightforward language feature, whose intended use is to facilitate function template argument deduction, has some rather surprisingly effective applications in template metaprogramming.6 November 2002: Expanding Monostate Protopattern
We discuss the use of a template member function in a non-template class to implement an extended version of the Monostate pattern. This version of Monostate allows the seamless addition and removal of Monostate members (at compile time) simply by referencing them.Note: the items on C++ gotchas that appeared in this space prior to November 2002 are now available in book form as C++ Gotchas.