STEVE DEWHURST | C++
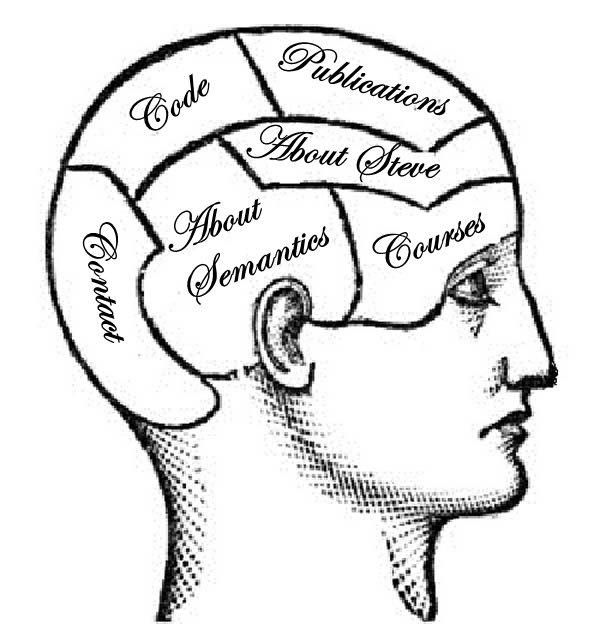
Code
This page contains C++ code from a variety of sources:- Complete source for my "Common Knowledge" column that appeared in The C/C++ Users Journal or in CUJ's "Online Experts" forum.
- Source that accompanied this site's (currently inactive) "Once, Weakly" web column.
- Stuff I've been thinking about.
Contents
- Compile-time type manipulation
- typeof operator implementations
- STL iterators
- STL containers
- Extended precision compile-time arithmetic
- original typeint implementation
- typeint multiplication and optimized multiplication
- n-ary typeint implementation
- different approach in original typeof implementation
- Patterns
Type Dismantling
A framework for the dismantling, pattern matching/substitution, and regeneration of a type. See a description of the framework in the Once, Weakly section of this site.dismantle.h
Typelist Meta-Algorithms
Algorithms, meta-function objects, and meta-function object adapters for manipulating typelists. See a decription of the techniques in the Once, Weakly section of this site.This code has been updated, as described in another Once, Weakly
typelist.h
utils.h
metafunction.h
typlistalgorithms.h
main.cpp
Named Expanding Monostate protopattern
This is a technique in search of an application. See a description of the technique in the Once, Weakly section of this site.expandingmonostate.cpp
Typeints
CUJ 21(6), June 2003: "N-Ary Nibbling"Moving typeints from a binary representation to a 2**n representation in order to deal with compilers' restrictive template instantiation recursion depth limits. Compile time arithmetic in excess of 5000-bit precision is possible.
typeint_nary.h
utils.h
main.cpp
CUJ 21(4), April 2003: "Scouting
Out an Optimization"
A detailed examination of a typeint multiplication algorithm, and
discussion
of metaprogramming techniques for improving its compile time.
typeint_mul.h
utils.h
main.cpp
CUJ
21(2), February 2003: "Typeints"
This facility is the first version of a library that performs
extended-precision
compile time arithmetic using the type system instead of binary
arithmetic.
Depending on the capabilities of the compiler, it's possible to perform
compile time arithmetic on 1000-bit (or larger) integers.
The first set of files
consists of a simple version of the typeint
facility
and a test program.
typeint.h
utils.h
main.cpp
The
second set of files is a
reimplementation
of the typeof operator implementation (see below) using typeints to
perform
the extended-precision compile time arithmetic.
gengn.h
gentype.h
typeof.h
typeint.h
utils.h
Typeof
CUJ 20(8), August 2002: "A Bit-Wise Typeof Operator, Part 1"CUJ 20(10), October 2002: "A Bit-Wise Typeof Operator, Part 2"
CUJ 20(12), December 2002: "A Bit-Wise Typeof Operator, Part 3"
Here is the source code for the of the typeof implementation that translates a type into a Gödel number, then back to a type in order to implement most of the functionality of a built-in typeof operator. It includes some elementary type manipulation utilities, the multi-integer compile time shift operations, the Gödel number generator, and the Gödel number to type regeneration. The code is almost identical to that which appeared in the article, but has been factored somwhat to make it cleaner, and (secondarily) make it compile a little faster.
utils.h
multishift.h
gengn.h
gentype.h
typeof.h
CUJ 20(8), August 2002:
"A Bit-Wise Typeof Operator,
Part
1"
There was a bug in one of the code examples. I had this
to say about it in the December, 2002 CUJ.
List
CUJ 20(6), June 2002: "Running Circles Round You, Logically": ListList (great name!) is an STL-like container that uses two-way pointers to implement a singly-linked list that can change its mind as to which way it's organized. Its iterators are similarly confused, and this makes for some interesting complexity results.
Note: A problem with the use of ptrdiff_t to hold a pointer value has been fixed. Use list2.h in preference to the original list.h.
Note 2: See flist, below, for a better altenative to List.
list2.h
flist
flist is an STL-compliant version of List. flist uses 2-way pointers to implement a container that has the same members and performance as std::list, but that uses half the working storage. It also provides fickle-bidirectional iterators and a constant time reverse operation.flist.h
flist documentation
MultiIn
CUJ 20(4), April 2002: "Metaprogrammed Adapters": MultiInMultiIn is an input/forward iterator adapter that allows an unbounded number of differently-typed input/forward sequences to be treated as a single "supersequence," without copying the values from the constituent sequences.
multiin.h
MultiOut
CUJ 20(2), February 2002: "Output Iterator Adapters": MultiOutMultiOut is an output iterator adapter that allows an output sequence to be sent to an unbounded number of output streams simultaneously.
multiout.h